Panthers detection engine can make API calls out to third-party resources such as enrichment providers or threat intelligence feeds. While the majority of enrichment use cases can be achieved using custom or built-in Lookup Tables, Panther can support this workflow by granting special permissions to the detection engine that runs your Python code and allowing you access to secrets stored in your own AWS account.
To create and store secrets in AWS Secrets Manager, please reference the AWS documentation: Create an AWS Secrets Manager secret.
Panthers detection engine runs within an AWS Lambda over every single log event as its received. To understand this level of scale, if you are processing one million log events in an hour, and if you are making an API call in even half of those detections, it will very quickly exacerbate any API rate limits that may be in place and likely affect detection performance.Because of this, its crucial to implement third-party API calls strategically and deliberately in Panther detections. See the Tips section below for implementation ideas.
Create a custom IAM role
AWS documentation reference: Creating IAM policies.
To access your stored secrets, Panther needs an IAM role with permissions to read from Secrets Manager. Create an IAM role following the steps below.
Navigate to IAM
Click Create role
Select Custom trust policy (see: Add trust policy)
Add the required permissions
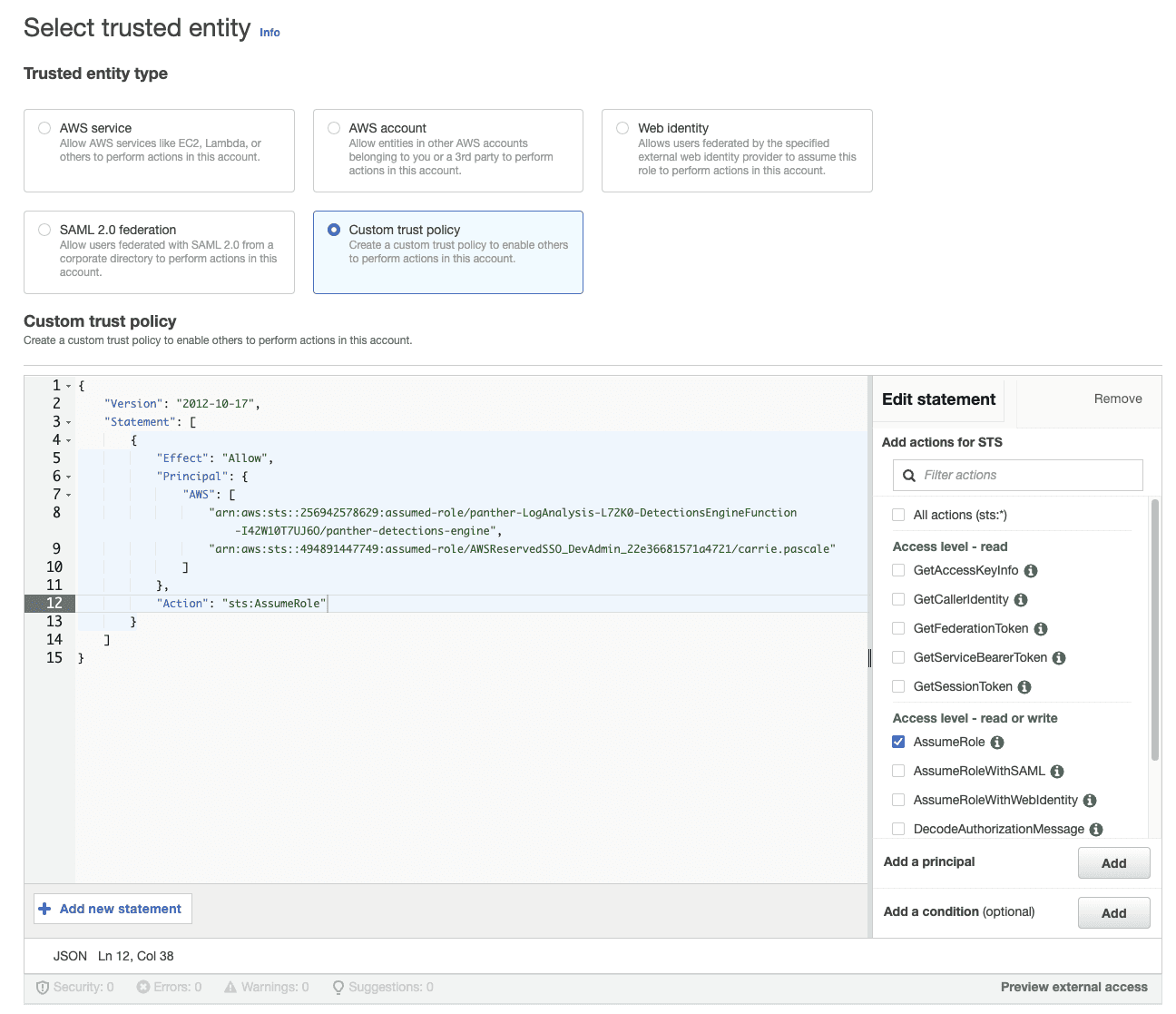
Click Next
Select Create policy
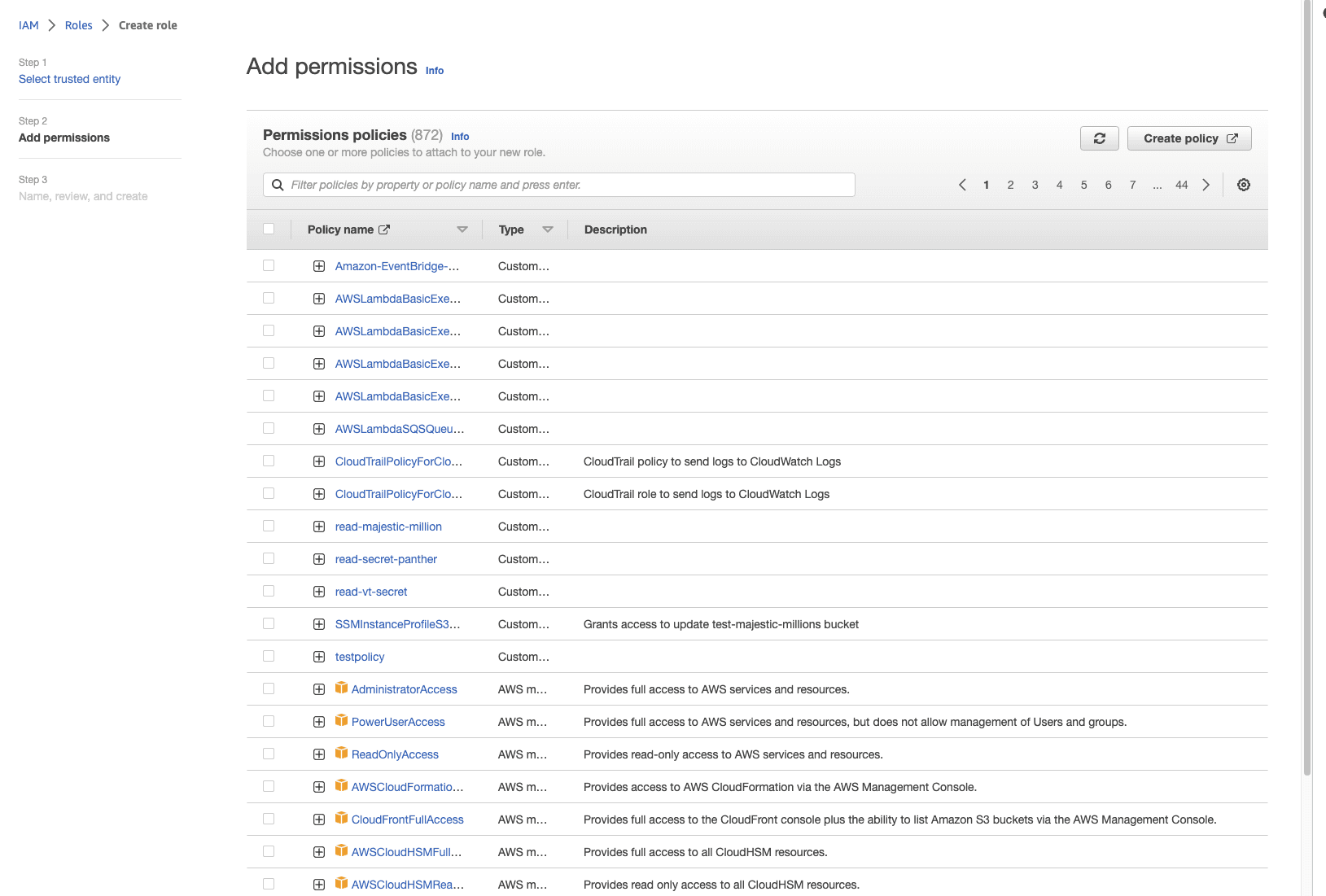
Under Specify permissions, add
GetSecretValue
andDescribeSecret
(see: Add IAM permissions)
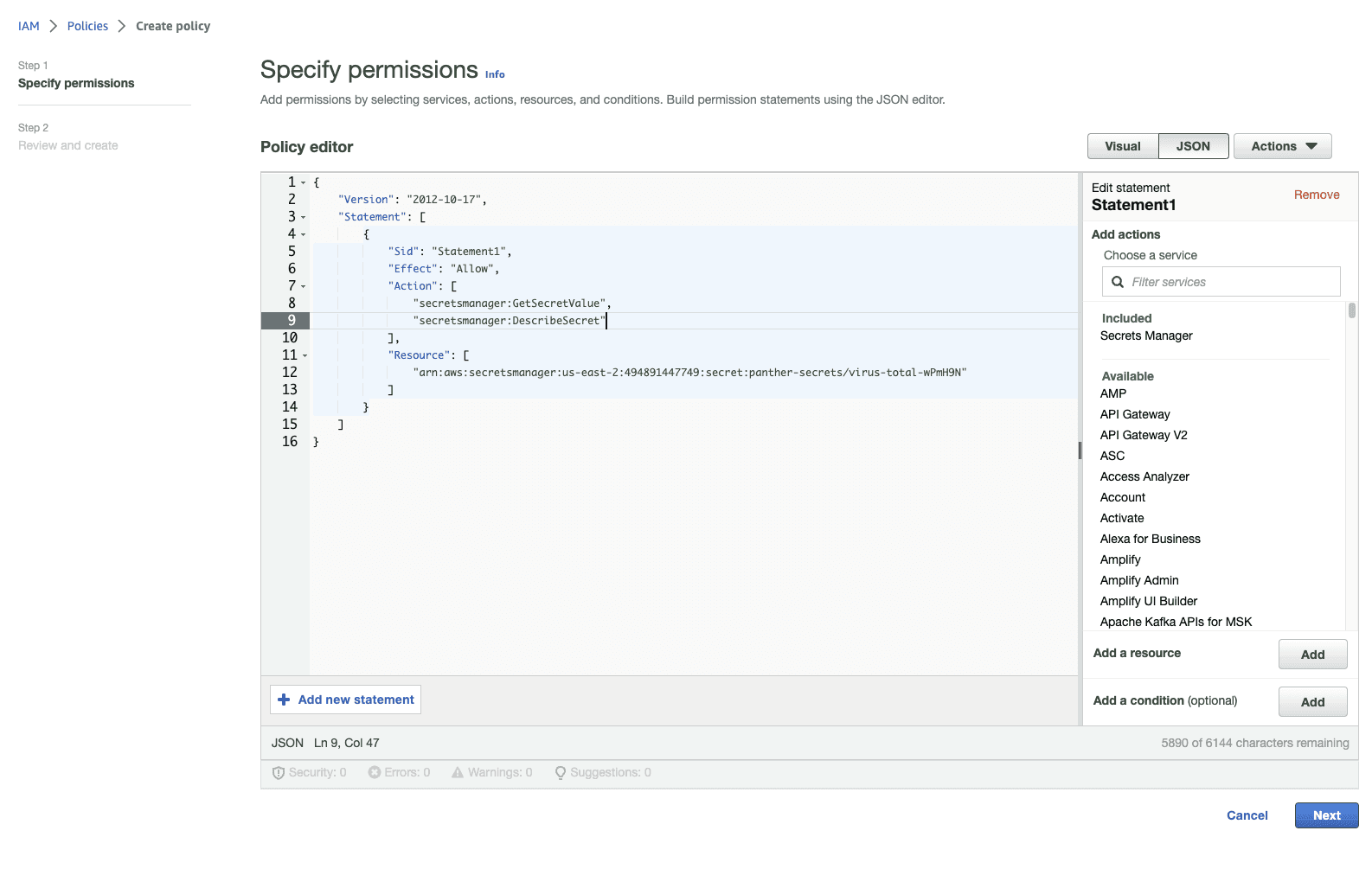
Give your policy a name and description
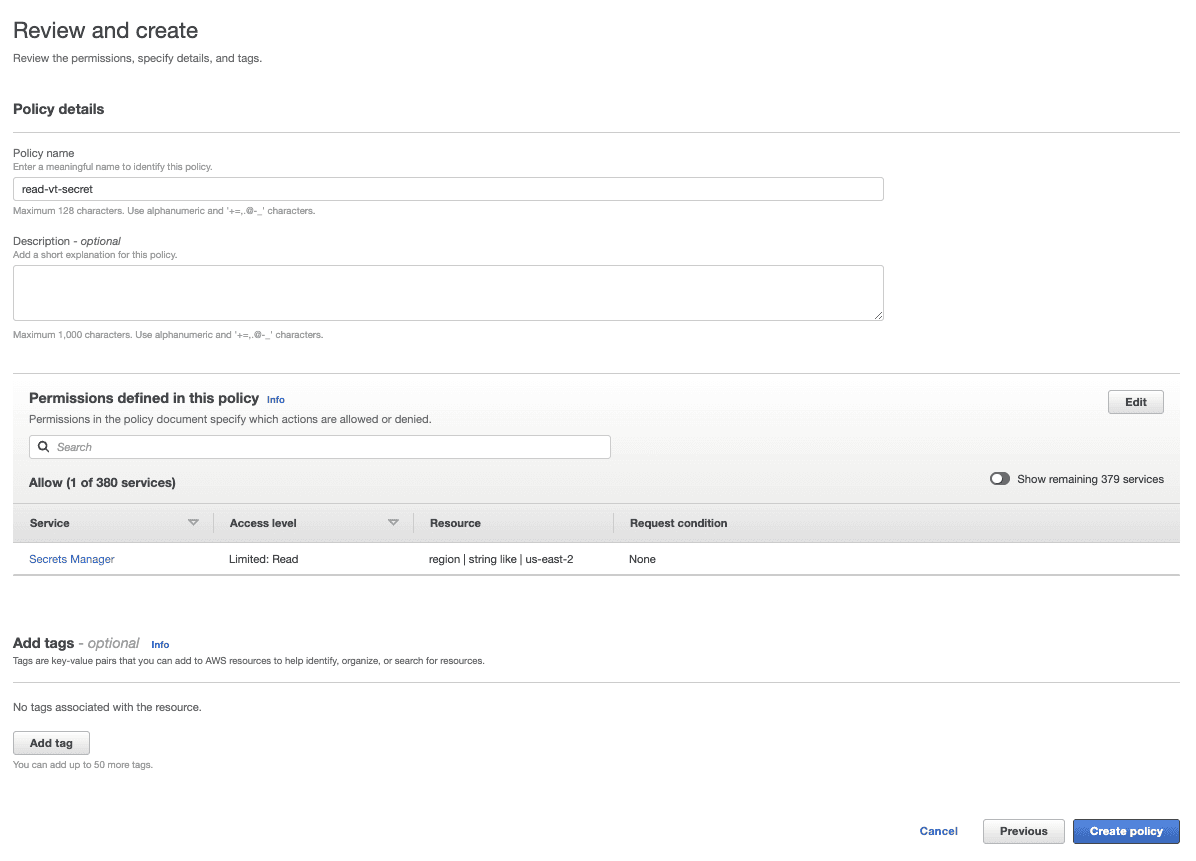
Navigate back to your role
In the Add permissions page, find and select your new policy (you may need to click the refresh button)
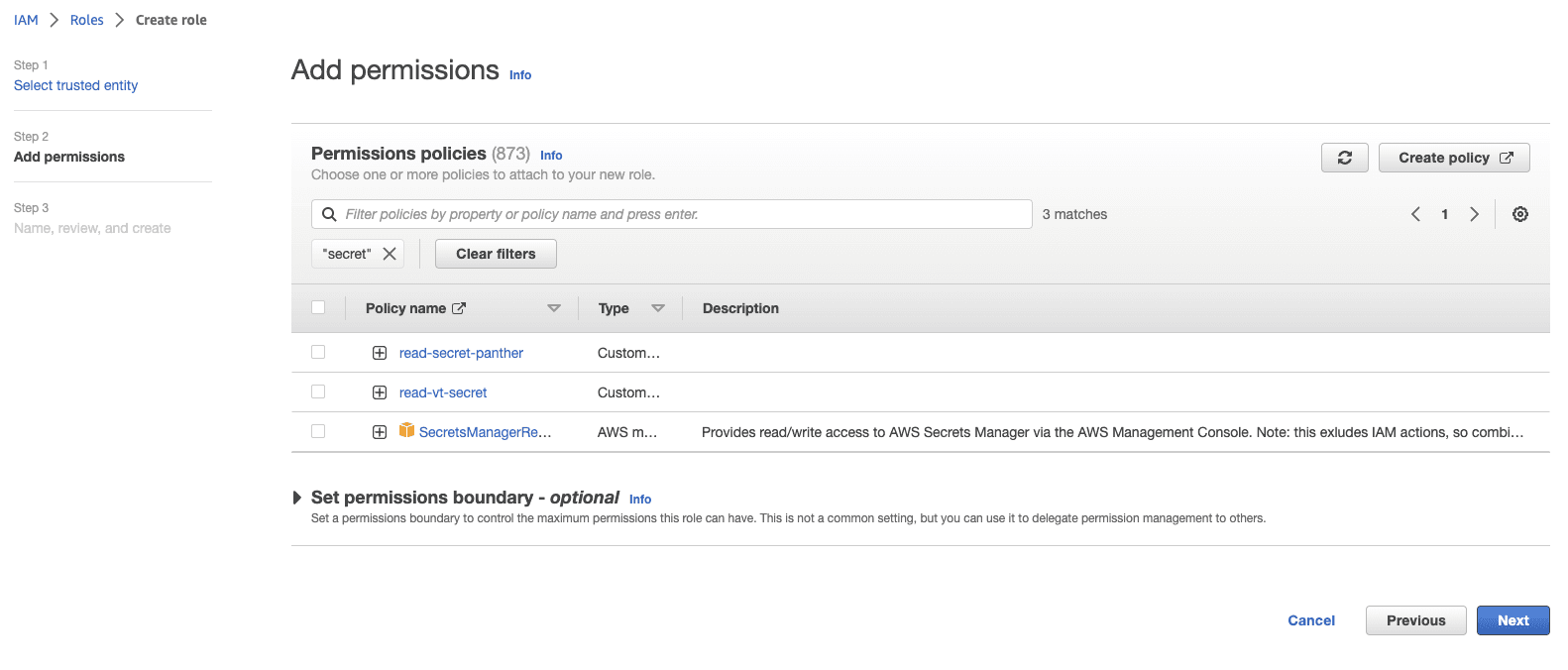
Select Create role
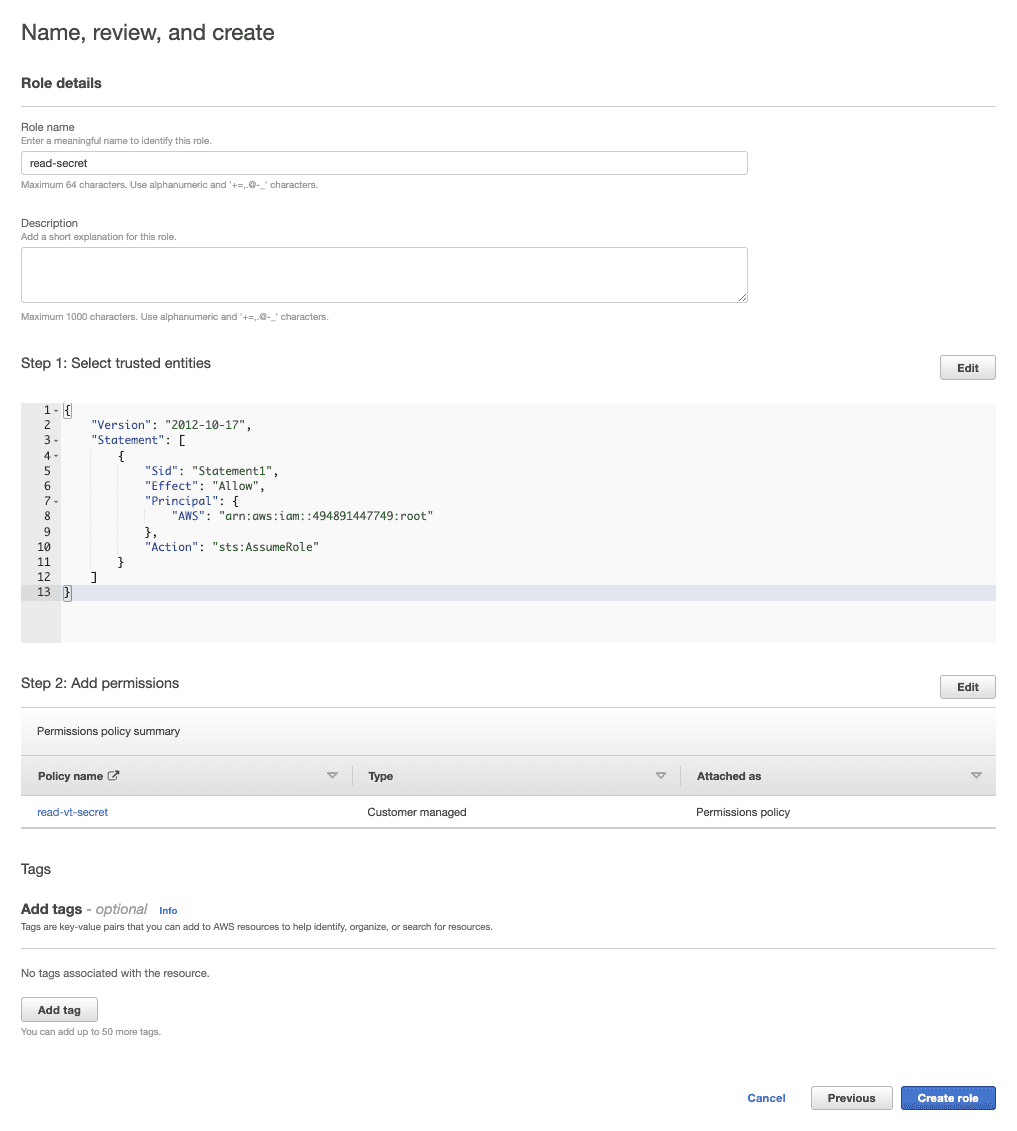
Copy the Role ARN
Add trust policy
In order to explicitly grant Panther the necessary permissions to access your secret, you need to set the Principal(s) that are allowed to assume your role. In this case, you will grant role-based access to the Panther detections engine.
You will need the role ARN used to execute the Panther detections engine. If you want to access your secret while testing, you will need to grant access to a second role used for running unit tests.
Please reach out to your Panther team to request these two ARNs. You cannot move forward without them.
Once youve received the required detections engine role ARN and optionally, the unit tests role ARN from Panther, add them as Principals to your trust policy. It should look like this:
Add IAM permissions
Your IAM policy must include these statements: GetSecretValue
and DescribeSecret
. As a best practice, it is recommended to only allow Panther access to the required secret for your API calls.
Your IAM policy should look like this:
Finish the configuration
After you have successfully created your IAM role, provide the role ARN you copied in Step 12 to your Panther team. They will add it to a backend deployment task which creates a list of roles that the detection engine has permission to assume. Once this step has been completed, you can proceed to writing detections.
Writing detections using stored secrets
To use your secret within a Panther detection, you can first write code that retrieves the secret, and then use it to make calls to a third-party API. boto3
and requests
are two Python libraries that are installed out-of-the-box in your Panther runtime environment, and should be used for this use case.
Rather than writing logic in each rule definition to assume your custom role, you can write one helper module and import it into multiple detections. Using boto3
, you can instantiate STS and Secrets Manager clients, assume the role programmatically via the assume_role method, and then fetch the secret from Secret Manager via the get_secret_value method.
Your helper might look like this:
Once you have defined code that retrieves the necessary credentials and fetches the secret value off of the returned credentials object, you can use the requests
library to call the API.
The example below looks up an IP address report from Virus Total. The alert_context function makes a call out to Virus Total and returns a last_analysis_stats
object. It uses the get_aws_credentials
and get_stored_secret
helpers defined above to fetch a secret named panther-secrets/virus-total. It also checks the IP address on the event using Panthers unified data model syntax, meaning this detection can be easily configured to run over multiple log types.
Tips
Before implementing a detection with an API call, explore Panthers built-in enrichment providers: GreyNoise, Tor Exit Nodes and IPInfo. These datasets come out-of-the-box with Panther and may already have the extra context you need to leverage.
For large, static datasets, or when you want to enrich log events with context, create a custom Lookup Table instead.
See: Lookup Tables.
Use filtering logic and caching helpers to store values in dynamoDB and reduce the number of API calls needed.